Debes crear botones dinamicamente, usando un layout personalizado para tu botón, también puedes modificar las propiedades del mismo según lo requieras, por ejemplo puedes modificar el texto, su color, el icono, incluso su posición...
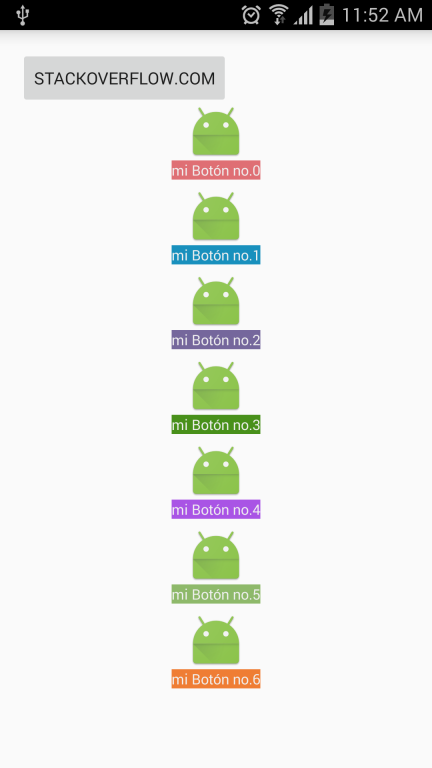
Este sería un código que demuestra lo que comento:
LinearLayout btnsContainer = new LinearLayout(getApplicationContext());
btnsContainer.setLayoutParams(new LinearLayout.LayoutParams(TableLayout.LayoutParams.MATCH_PARENT, TableLayout.LayoutParams.WRAP_CONTENT));
btnsContainer.setOrientation(LinearLayout.VERTICAL);
btnsContainer.setGravity(Gravity.CENTER);
//Crea botons dinamicamente.
for (int i = 0; i < 100; i++){
final LinearLayout buttonContainer = (LinearLayout) LayoutInflater.from(getApplicationContext()).inflate(R.layout.item_button,null);
ImageView btnImg = (ImageView) buttonContainer.findViewById(R.id.btn_image);
TextView btnTxt = (TextView) buttonContainer.findViewById(R.id.btn_text);
btnTxt.setText("mi Botón no." + i);
btnTxt.setBackgroundColor(getRandomColor());
btnImg.setImageResource(R.mipmap.ic_launcher);
buttonContainer.setTag(i);
buttonContainer.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), " Listener botón " + v.getTag() , Toast.LENGTH_SHORT).show();
}
});
//Va agregegando botones al contenedor.
btnsContainer.addView(buttonContainer);
}
//Crea contenedor para agregar contenedor de botones.
FrameLayout.LayoutParams paramsContainer = new FrameLayout.LayoutParams(400, 1500, Gravity.CENTER);
//Agrega contenedor con botones.
addContentView(btnsContainer, paramsContainer);
Función para obtener colores aleatorios:
public int getRandomColor(){
Random rnd = new Random();
return Color.argb(255, rnd.nextInt(256), rnd.nextInt(256), rnd.nextInt(256));
}
Este sería el layout del botón personalizado item_button.xml
:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="10px"
android:orientation="vertical" >
<ImageView
android:id="@+id/btn_image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:contentDescription="@null"/>
<TextView
android:id="@+id/btn_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="12sp"
android:textColor="#F0F0F0" />
</LinearLayout>